Westermania Documentation
Introduction
The Aura Scenegraph allows a 3D-Scene to be modelled in a tree structure, allowing
efficient organisation, transformations, culling, collision-detection, etc.
Scenegraph structure
An Aura Scenegraph is a tree (a directed acyclic graph), in witch all nodes are objects
of the class caGraph or a subclass of caGraph.
The tree's root element is a caScene object, the inner nodes are
caGraph objects. All geometry, lighting, etc. information is stored
in the leaf-nodes, which can be objects of the following classes:
caGeometry, caLight, or caCamera.
Example of the Scenegraph structure
The scene's geometry (Points, Triangles, Polygons, etc.) and it's respective state (color,
texture, fill-mode, etc.) are stored in the caGeometry nodes, light sources
(directional, ambient, etc.) are stored in caLight nodes, and cameras (mobile
viewports) are stored in caCamera nodes.
Geometry organization
To make use of the advantages of the scenegraph, the scene's geometry should be organised
efficiently.
In general, smaller objects that are attached to (or belong to) a larger object (e.i. fingers
and a hand) should be in a child-graph of the graph the large object is stored in. This not
only allows for efficient culling etc. (if the hand isn't in sight, the fingers needn't be
checked) it also enables efficient and natural movement. Any transformation applied to the hand
will also be applied to the fingers, however moving the fingers won't move the hand!
Transformations (scaling, rotation, etc.) are stored in any caGraph object. They
are applied to all child-nodes, or to the object itself, if it is a leaf node.
Example of graph organisation
Describing Geometry
All of the scene's actuall geometry, triangles, polygons, etc., are stored
in buffers, (objects of the class caVertexBuffer).
Vertex buffers store a list of vertices (x,y,z coordinates), and a variable specifying the type
of geometry that the vertices represent (lines, triangles, quads, etc.).
Vertex buffers only store pure geometry. Information on how the geometry should be rendered
(color, texture, etc.) are stored in state buffers (caState). State buffers
hold information for everything from filling modes over color and textures to lighting of
geometry.
Every caGeometry-node owns (is assigned a) vertex buffer, and a state buffer. Only
one vertex and state buffer can be active in a geometry-node at any given time, however
vertex and state buffers can be assigned to (owned by) several geometry-nodes at once.
In other words indentical geometry doesn't have to be stored several times and geometry with
the same states don't have to be described seperately.
Example of a complete scene graph
Aura Class-Hierachy:
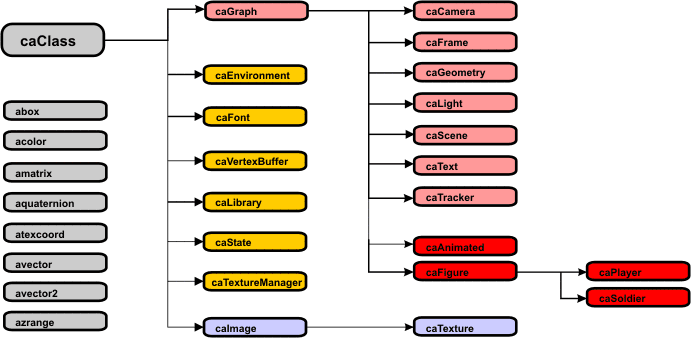
Vertex Buffers
Description coming soon. See comments in Sourcecode for first Information.
State Buffers
Description coming soon. See comments in Sourcecode for first Information.
Geometry-Nodes
The caGeometry class, is a leaf-node of the scene Graph. It contains
geometry (using a vertex buffer), the geometry's state (using a state
buffer), and transformations to be performed on the contained geometry.
The constructor takes no arguments.
Methods:
.......................
Light-Nodes
The caLight class, is a leaf-node of the scene Graph. It contains informtion
about the ambien-, diffuse-, specularcolor (and the position ???).
The constructor takes no arguments.
Methods:
- public virtual void
Render ( int stage ) ;
recursive render traversal function (called internally)
@param stage render stage (setup, z-buffer or no z-buffer)
- void Ambient (float
r,float g,float
b,float a) ;
set ambient color from byte components (like glColor4ub)
paramaters range from 0 to 255
- void Ambient(uchar
r,uchar g,uchar
b,uchar a) ;
set ambient color from byte components (like glColor4ub)
parameters range from 0 to 255
- void Ambient(int
rgba) ;
set ambient color from packed signed integer
@param rgba packed color (as 0xRRGGBBAA)
- void Ambient(uint
rgba) ;
same as above with unsigned integer
- void Ambient(float*
rgba) ;
set ambient color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void Ambient(uchar*
rgba) ;
set ambient color from byte array (like glColor4ubv)
@param rgba array of byte color components (between 0 and 255) red, green,
blue and alpha
- void Ambient(acolor
c) ;
returns ambient color
- void Diffuse(float
r,float g,float
b,float a) ;
set diffuse color from float components (like glColor4f)
parameters range from 0.0 to 1.0
- void Diffuse(uchar
r,uchar g,uchar
b,uchar a) ;
same as above with parameter-range from 0 to 255
- void Diffuse(int
rgba) ;
set diffuse color from packed signed integer
this avoids problems with compiler ambiguities. A 0 will be treated as the
packed version of transparent black.
@param rgba packed color (as 0xRRGGBBAA)
- void Diffuse(uint
rgba) ;
set diffuse color from packed unsigned integer
@param rgba packed color (as 0xRRGGBBAA)
- void Diffuse(float*
rgba) ;
set diffuse color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void Diffuse(uchar*
rgba) ;
same like above with a byte arrray and values from 0 to 255
- void Diffuse(acolor
c);
returns diffuse color
- void Specular(float
r,float g,float
b,float a) ;
set specular color from float components (like glColor4f)
r,g,b,a range from 0.0 to 1.0
- void Specular(uchar
r,uchar g,uchar
b,uchar a) ;
same thing with values from 0 to 255
- void Specular(int
rgba) ;
set specular color from packed signed integer
this avoids problems with compiler ambiguities. A 0 will be treated as the
packed version of transparent black.
@param rgba packed color (as 0xRRGGBBAA)
- void Specular(int
rgba) ;
same as above with unsignded integer values
- void Specular(float*
rgba) ;
set specular color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void Specular(uchar*
rgba) ;
set specular color from byte array (like glColor4ubv)
@param rgba array of byte color components (between 0 and 255) red, green,
blue and alpha
- void Specular(acolor
c) ;
set specular color from an acolor
Camera-Node
The caCamera class, is a leaf-node of the scene Graph.It holds information
about the field of view, H/O aspect ratio, deph-range and the projectionmatrix.
The constructor takes no arguments.
Methods:
- void setViematrix() ;
- void set ProjectionMatrix() ;
- void FOV(float
angle) ;
set horizontal field-of-view
@param angle horizontal field-of-view angle (degrees)
- float FOV() ;
returns horizontal field-of-view
- void Aspect(float aspect) ;
set H/V aspect ratio
- float Aspect() ;
returns the H/V aspect ratio
- void Range(float
n,float f)
set depth range using floats
@param n near depth distance (should be positive)
@param f far depth distance (should be positive)
- void Range(azrange
nf) ;
set depth range using an azrange
@param nf near-far arange
- azrange Range();
returns depth range
The Scene-Node
Methods:
- virtual void Render(int
stage) ;
recursive render traversal function (called internally) ;
@param stage render stage (setup, z-buffer or no z-buffer)
- void Background(float
r,float g,float
b,float a) ;
set background color from byte components (like glColor4ub)
paramaters range from 0 to 255
- void Background(int
rgba) ;
set background color from packed signed integer
this avoids problems with compiler ambiguities. A 0 will be treated as the
packed version of transparent black.
@param rgba packed color (as 0xRRGGBBAA)
- void Background(uint
rgba)
same as above, but with unsigned integer as parameter
- void Background(float*
rgba) ;
set background color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void Background(uchar*
rgba) ;
set background color from byte array (like glColor4ubv)
@param rgba array of byte color components (between 0 and 255) red, green,
blue and alpha
- void Background(acolor
c) ;
set background color from an acolor
- acolor Background() ;
returns background color
- void Ambient(float
r,float g,float
b,float a) ;
set global ambient color from float components (like glColor4f)
paramters range from 0.0 to 1.0
- void Ambient(uchar
r,uchar g,uchar
b,uchar a) ;
same as above but values from 0 to 255
- void Ambient(int
rgba) ;
set global ambient color from packed signed integer
this avoids problems with compiler ambiguities. A 0 will be treated as the
packed version of transparent black.
@param rgba packed color (as 0xRRGGBBAA)
- void Ambient(uint
rgba) ;
same as above with unsigned integer
- void Ambient(float*
rgba) ;
set global ambient color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void Ambient(uchar*
rgba) ;
same as above with parametervalues rangin from 0 to 255
- void Ambient(acolor
c) ;
set global ambient color from an acolor
- acolor Ambient() ;
returns global ambient color
- void FogColor(float
r,float g,float
b,float a) ;
set fog color from float components (like glColor4f)
parameterrange: 0.0 -1.0
- void FogColor(uchar
r,uchar g,uchar
b,uchar a) ;
same as above but with values from 0 to 255
- void FogColor(int
rgba) ;
set fog color from packed signed integer
this avoids problems with compiler ambiguities. A 0 will be treated as the
packed version of transparent black.
@param rgba packed color (as 0xRRGGBBAA)
- void FogColor(uint
rgba) ;
see above and use unsigned integer values
- void FogColor(float*
rgba) ;
set fog color from float array (like glColor4fv)
@param rgba array of floating point color components (between 0.0 and 1.0)
red, green, blue and alpha
- void FogColor(uchar*
rgba) ;
look above and use parameter values form 0 to 255
- void FogColor(acolor
c) ;
set fog color from an acolor
- acolor FogColor();
returns fog color
- void FogDensity(float
density) ;
sets fog density
@param density fog density (exp-square algorithm)
- float FogDensity();
returns fog density
- void Camera(caCamera*
camera) ;
select camera in the scene
@param camera camera to select (must be a graph in the same scene)
- caCamera* Camera() ;
returns current active camera
caGraph Class